In this example we will display the price in USD of 1 bitcoin, the data is taken from coindesk’s API and displayed on an oled display.
This is similar to our previous examples, you do not need to use a Wemos mini or the OLED shield below but this makes the project easier to build
Here is the oled shield for the Wemos mini
Here is the wemos mini
Code
#include <ESP8266WiFi.h> #include <Wire.h> #include <SFE_MicroOLED.h> #include <ArduinoJson.h> const char* ssid = "iainhendry"; const char* pass = "iain061271"; #define PIN_RESET 255 // #define DC_JUMPER 0 // I2C Addres: 0 - 0x3C, 1 - 0x3D String id; String value; String json; MicroOLED oled(PIN_RESET, DC_JUMPER); // I2C Example WiFiClient client; // delay between updates const unsigned long postingInterval = 60L * 1000L; unsigned long lastConnectionTime = 0; void setup() { delay(100); Serial.begin(115200); Serial.println(); Serial.println(); Serial.print("Connecting to "); Serial.println(ssid); WiFi.begin(ssid, pass); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println(""); Serial.println("WiFi connected"); Serial.println("IP address: "); Serial.println(WiFi.localIP()); Serial.print("Connecting to "); oled.begin(); oled.clear(ALL); oled.setCursor(0,0); oled.display(); oled.clear(PAGE); oled.clear(ALL); oled.print("Bitcoin price"); oled.setCursor(0,1); oled.print("loading.."); oled.display(); // Display what's in the buffer (splashscreen) delay(50); } int check_connect = 0; void httpRequest() { client.stop(); // if there's a successful connection: if (client.connect("api.coindesk.com", 80)) { Serial.println("connecting..."); client.println("GET /v1/bpi/currentprice.json HTTP/1.1"); client.println("Host: api.coindesk.com"); client.println("User-Agent: ESP8266/1.1"); client.println("Connection: close"); client.println(); lastConnectionTime = millis(); } else { // if you couldn't make a connection: Serial.println("connection failed"); } } void loop() { int cnt; if (cnt++ == 10000) { cnt = 0; if (check_connect++ == 50) { check_connect = 0; if (WiFi.status() != WL_CONNECTED) { } } } if (millis() - lastConnectionTime > postingInterval) { httpRequest(); unsigned int i = 0; //timeout counter int n = 1; // char counter char json[500] ="{"; while (!client.find("\"USD\":{")){} while (i<20000) { if (client.available()) { char c = client.read(); json[n]=c; if (c=='}') break; n++; i=0; } i++; } StaticJsonBuffer<500> jsonBuffer; JsonObject& root = jsonBuffer.parseObject(json); String newjson = root["code"]; String value = root["rate"]; id = newjson.substring(9,12); // value = newjson.substring(41,51); oled.display(); oled.clear(PAGE); // Clear the display's internal memory oled.clear(ALL); // Clear the library's display buffer oled.setCursor(0,1); oled.print(value); oled.display(); id=""; value=""; } }
testing
You should see something like this on the oled display
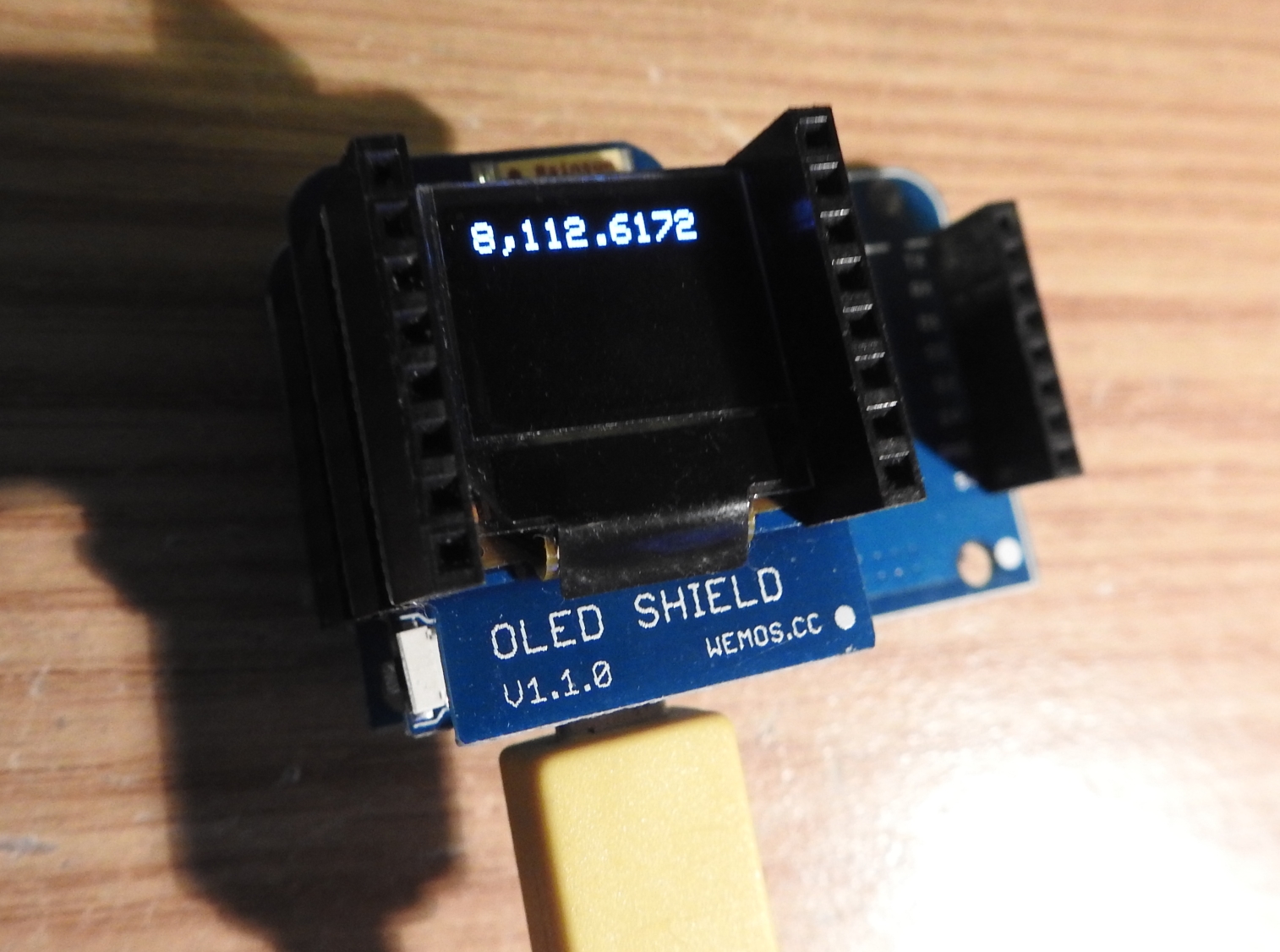
Links
Here are some of the products I used
by hotcoin.net
Looks like there is a memory leak somewhere as the sketch stops after about 95 – 100 iterations.